在Python中獲取數據可以透過多種方式完成,具體取決於數據的來源和格式。以下是一些常見的數據獲取方法和庫:
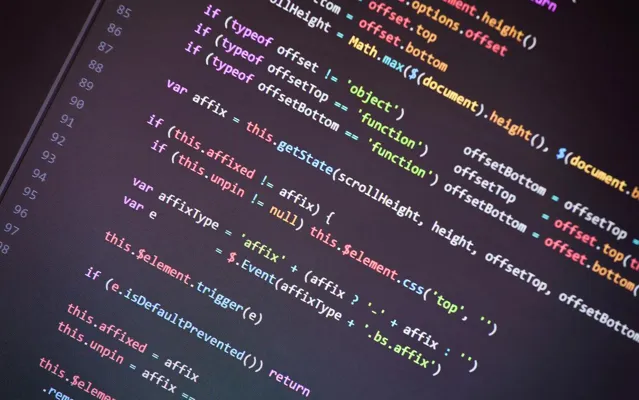
請點選輸入圖片python獲取數據的方法描
1. 從檔中讀取數據
文字檔案
使用內建的open()函數讀取文字檔案。
使用read(), readline(), readlines()等方法讀取數據。
python
with open('data.txt', 'r') as file:
data = file.read() # 讀取整個檔
# 或者
lines = file.readlines() # 讀取所有行到列表中
CSV檔
使用csv模組讀取CSV檔。
python
import csv
www.baijingpt.com/
with open('data.csv', 'r') as csvfile:
reader = csv.reader(csvfile)
for row in reader:
print(row)
Excel檔
使用openpyxl(針對.xlsx)或xlrd(針對.xls)庫讀取Excel檔。
python
from openpyxl import load_workbook
wb = load_workbook(filename='data.xlsx')
sheet = wb.active
for row in sheet.iter_rows(values_only=True):
print(row)
2. 從網絡獲取數據
使用requests庫獲取網頁數據。
python
import requests
url = 'http://www.zazugpt.com/'
response = requests.get(url)
data = response.json() # 假設返回的是JSON格式的數據
print(data)
使用BeautifulSoup和requests庫解析HTML頁面數據。
python
from bs4 import BeautifulSoup
import requests
url = 'https://www.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 使用soup解析HTML,例如:
titles = soup.find_all('p')
for title in titles:
print(title.text)
3. 從數據庫獲取數據
使用sqlite3(輕量級數據庫)或pymysql(MySQL)、psycopg2(PostgreSQL)等庫從數據庫中獲取數據。
python
import sqlite3
conn = sqlite3.connect('example.db')
c = conn.cursor()
# 查詢數據
c.execute('SELECT * FROM some_table')
rows = c.fetchall()
for row in rows:
print(row)
conn.close()
4. 使用API獲取數據
大多數現代數據服務都提供API介面,你可以使用requests等庫來呼叫這些API並獲取數據。
5. 即時數據流
對於即時數據流,如股票數據、社交媒體數據等,可能需要使用專門的庫或框架,如Tweepy(用於Twitter數據)、kafka-python(用於Apache Kafka)等。